Get - RequestParam String
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* RequestParam String으로 파라미터 받기
http://localhost:8080/example/parameter/example1?id=test123&code=B123
*/
@GetMapping("/example1")
public void example1(@RequestParam String id, @RequestParam String code, Model model) {
model.addAttribute("id", id);
model.addAttribute("code", code);
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${code}</h2>
</body>
</html>
- 결과
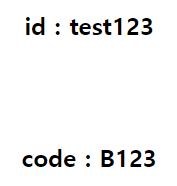
Get - RequestParam Map
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* RequestParam Map으로 파라미터 받기
http://localhost:8080/example/parameter/example2?id=test123&code=B123
*/
@GetMapping("/example2")
public void example2(@RequestParam Map<String, Object> paramMap, Model model) {
model.addAttribute("paramMap", paramMap);
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${paramMap.id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${paramMap.code}</h2>
</body>
</html>
- 결과
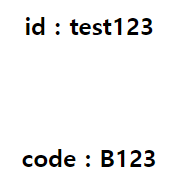
Get - Class
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* Class로 파라미터 받기
http://localhost:8080/example/parameter/example3?id=test123&code=B123
*/
@GetMapping("/example3")
public void example3(ExampleParameter parameter, Model model) {
model.addAttribute("parameter", parameter);
}
}
@Data
public class ExampleParameter {
private String id;
private String code;
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${parameter.id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${parameter.code}</h2>
</body>
</html>
- 결과
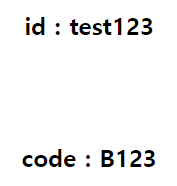
Get - PathVariable
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* PathVariable로 파라미터 받기
http://localhost:8080/example/parameter/example4/test123/B123
*/
@GetMapping("/example4/{id}/{code}")
public String example4(@PathVariable String id, @PathVariable String code, Model model) {
model.addAttribute("id", id);
model.addAttribute("code", code);
return "/example/parameter/example4";
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${code}</h2>
</body>
</html>
- 결과
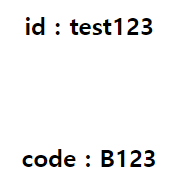
Get - String[ ]
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* String[]로 파라미터 받기1
http://localhost:8080/example/parameter/example5?ids=12&ids=34&ids=56
*/
@GetMapping("/example5")
public String example5(@RequestParam String[] ids, Model model) {
model.addAttribute("ids", ids);
return "/example/parameter/example5";
}
}
또는
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* String[]로 파라미터 받기2
http://localhost:8080/example/parameter/example5?ids=12&ids=34&ids=56
*/
@GetMapping("/example5")
public String example5(HttpServletRequest request, Model model) {
model.addAttribute("ids", request.getParameterValues("ids"));
return "/example/parameter/example5";
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">ids : ${ids}</h2>
<c:forEach var="id" items="${ids}">
<p>${id}</p>
</c:forEach>
</body>
</html>
- 결과
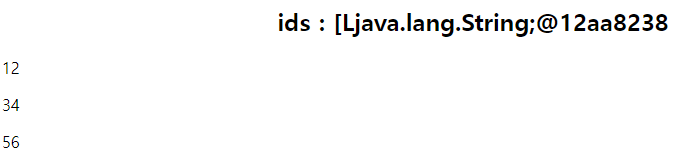
Post - RequestBody (JSON)
- 코드
get은 url에 파라메터가 노출되지만 post는 노출이안되고 body에 파라메터가 전송됨
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* JSON을 RequestBody로 받기
http://localhost:8080/example/parameter/example6/form
*/
@GetMapping("/example6/form")
public void form() {
}
@PostMapping("/example6/saveData")
@ResponseBody
public Map<String, Object> example6(@RequestBody Map<String, Object> requestBody) {
Map<String, Object> resultMap = new HashMap<String, Object>();
resultMap.put("result", true);
logger.info("requestBody : {}", requestBody);
return resultMap;
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script>
$(function() {
var json = {
user: {
name: '홍길동',
age: 14,
address: '대한민국'
}
};
console.log(json);
$.ajax({
url: '/example/parameter/example6/saveData',
type: 'post',
data: JSON.stringify(json),
contentType: 'application/json',
dataType: 'json',
success: function(data) {
console.log(data);
}
});
});
</script>
</body>
</html>
- 결과
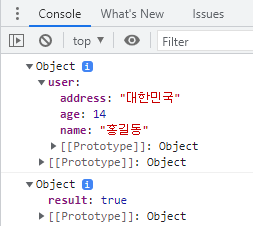
Post - Class (JSON)
- 코드
get은 url에 파라메터가 노출되지만 post는 노출이안되고 body에 파라메터가 전송됨
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* JSON을 Class로 받기
http://localhost:8080/example/parameter/example6/form
*/
@GetMapping("/example6/form")
public void form() {
}
@PostMapping("/example6/saveData")
@ResponseBody
public Map<String, Object> example6(@RequestBody ExampleRequestBodyUser requestBody) {
Map<String, Object> resultMap = new HashMap<String, Object>();
resultMap.put("result", true);
logger.info("requestBody : {}", requestBody);
return resultMap;
}
}
@Data
public class ExampleUser {
private String name;
private int age;
private String address;
}
@Data
public class ExampleRequestBodyUser {
private ExampleUser user;
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script>
$(function() {
var json = {
user: {
name: '홍길동',
age: 14,
address: '대한민국'
}
};
console.log(json);
$.ajax({
url: '/example/parameter/example6/saveData',
type: 'post',
data: JSON.stringify(json),
contentType: 'application/json',
dataType: 'json',
success: function(data) {
console.log(data);
}
});
});
</script>
</body>
</html>
- 결과
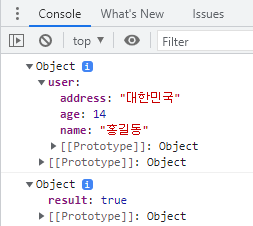
'Java-Spring > 자바 스프링부트 활용 웹개발 실무용' 카테고리의 다른 글
자바 스프링부트 활용 웹개발 실무용 - 목차 (0) | 2023.07.03 |
---|---|
[자바 스프링부트 활용 웹개발 실무용] 세션 관리를 Class 구조와 Spring을 활용하여 개발하기 (0) | 2022.09.15 |
[자바 스프링부트 활용 웹개발 실무용] 파일 업로드와 썸네일 생성 (0) | 2022.08.27 |
[자바 스프링부트 활용 웹개발 실무용] TypeHandler로 데이터 변환하기 (0) | 2022.08.20 |
[자바 스프링부트 활용 웹개발 실무용] cron으로 로컬, 개발, 운영 설정값 프로퍼티에 따른 스케줄러 사용 (0) | 2022.08.18 |
Get - RequestParam String
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* RequestParam String으로 파라미터 받기
http://localhost:8080/example/parameter/example1?id=test123&code=B123
*/
@GetMapping("/example1")
public void example1(@RequestParam String id, @RequestParam String code, Model model) {
model.addAttribute("id", id);
model.addAttribute("code", code);
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${code}</h2>
</body>
</html>
- 결과
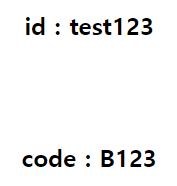
Get - RequestParam Map
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* RequestParam Map으로 파라미터 받기
http://localhost:8080/example/parameter/example2?id=test123&code=B123
*/
@GetMapping("/example2")
public void example2(@RequestParam Map<String, Object> paramMap, Model model) {
model.addAttribute("paramMap", paramMap);
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${paramMap.id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${paramMap.code}</h2>
</body>
</html>
- 결과
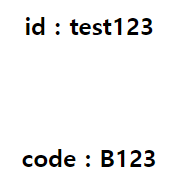
Get - Class
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* Class로 파라미터 받기
http://localhost:8080/example/parameter/example3?id=test123&code=B123
*/
@GetMapping("/example3")
public void example3(ExampleParameter parameter, Model model) {
model.addAttribute("parameter", parameter);
}
}
@Data
public class ExampleParameter {
private String id;
private String code;
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${parameter.id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${parameter.code}</h2>
</body>
</html>
- 결과
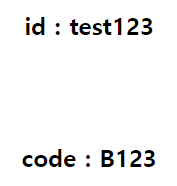
Get - PathVariable
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* PathVariable로 파라미터 받기
http://localhost:8080/example/parameter/example4/test123/B123
*/
@GetMapping("/example4/{id}/{code}")
public String example4(@PathVariable String id, @PathVariable String code, Model model) {
model.addAttribute("id", id);
model.addAttribute("code", code);
return "/example/parameter/example4";
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">id : ${id}</h2>
<h2 style="text-align: center; margin-top: 100px;">code : ${code}</h2>
</body>
</html>
- 결과
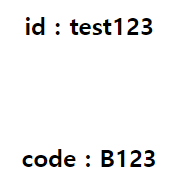
Get - String[ ]
- 코드
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* String[]로 파라미터 받기1
http://localhost:8080/example/parameter/example5?ids=12&ids=34&ids=56
*/
@GetMapping("/example5")
public String example5(@RequestParam String[] ids, Model model) {
model.addAttribute("ids", ids);
return "/example/parameter/example5";
}
}
또는
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* String[]로 파라미터 받기2
http://localhost:8080/example/parameter/example5?ids=12&ids=34&ids=56
*/
@GetMapping("/example5")
public String example5(HttpServletRequest request, Model model) {
model.addAttribute("ids", request.getParameterValues("ids"));
return "/example/parameter/example5";
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<h2 style="text-align: center; margin-top: 100px;">ids : ${ids}</h2>
<c:forEach var="id" items="${ids}">
<p>${id}</p>
</c:forEach>
</body>
</html>
- 결과
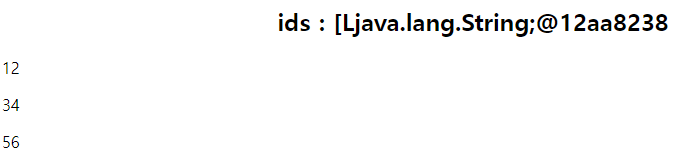
Post - RequestBody (JSON)
- 코드
get은 url에 파라메터가 노출되지만 post는 노출이안되고 body에 파라메터가 전송됨
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* JSON을 RequestBody로 받기
http://localhost:8080/example/parameter/example6/form
*/
@GetMapping("/example6/form")
public void form() {
}
@PostMapping("/example6/saveData")
@ResponseBody
public Map<String, Object> example6(@RequestBody Map<String, Object> requestBody) {
Map<String, Object> resultMap = new HashMap<String, Object>();
resultMap.put("result", true);
logger.info("requestBody : {}", requestBody);
return resultMap;
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script>
$(function() {
var json = {
user: {
name: '홍길동',
age: 14,
address: '대한민국'
}
};
console.log(json);
$.ajax({
url: '/example/parameter/example6/saveData',
type: 'post',
data: JSON.stringify(json),
contentType: 'application/json',
dataType: 'json',
success: function(data) {
console.log(data);
}
});
});
</script>
</body>
</html>
- 결과
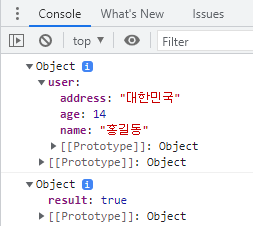
Post - Class (JSON)
- 코드
get은 url에 파라메터가 노출되지만 post는 노출이안되고 body에 파라메터가 전송됨
@Controller
@RequestMapping("/example/parameter")
public class ExampleParameterController {
Logger logger = LoggerFactory.getLogger(getClass());
/* JSON을 Class로 받기
http://localhost:8080/example/parameter/example6/form
*/
@GetMapping("/example6/form")
public void form() {
}
@PostMapping("/example6/saveData")
@ResponseBody
public Map<String, Object> example6(@RequestBody ExampleRequestBodyUser requestBody) {
Map<String, Object> resultMap = new HashMap<String, Object>();
resultMap.put("result", true);
logger.info("requestBody : {}", requestBody);
return resultMap;
}
}
@Data
public class ExampleUser {
private String name;
private int age;
private String address;
}
@Data
public class ExampleRequestBodyUser {
private ExampleUser user;
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script>
$(function() {
var json = {
user: {
name: '홍길동',
age: 14,
address: '대한민국'
}
};
console.log(json);
$.ajax({
url: '/example/parameter/example6/saveData',
type: 'post',
data: JSON.stringify(json),
contentType: 'application/json',
dataType: 'json',
success: function(data) {
console.log(data);
}
});
});
</script>
</body>
</html>
- 결과
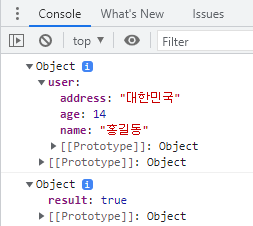
'Java-Spring > 자바 스프링부트 활용 웹개발 실무용' 카테고리의 다른 글
자바 스프링부트 활용 웹개발 실무용 - 목차 (0) | 2023.07.03 |
---|---|
[자바 스프링부트 활용 웹개발 실무용] 세션 관리를 Class 구조와 Spring을 활용하여 개발하기 (0) | 2022.09.15 |
[자바 스프링부트 활용 웹개발 실무용] 파일 업로드와 썸네일 생성 (0) | 2022.08.27 |
[자바 스프링부트 활용 웹개발 실무용] TypeHandler로 데이터 변환하기 (0) | 2022.08.20 |
[자바 스프링부트 활용 웹개발 실무용] cron으로 로컬, 개발, 운영 설정값 프로퍼티에 따른 스케줄러 사용 (0) | 2022.08.18 |